Unittest Runner
Navigation
- Unit Test Runner Javascript
- Stackoverflow.com › Questions › 307357Best Test Runner? (Unit Testing, .NET) - Stack Overflow
- .net Core - Dotnet Test Command To Display Individual Test ..
- See Full List On Github.com
- modules |
- next |
- previous |
- PyMOTW »
- Development Tools »
Purpose: | Automated testing framework |
---|---|
Available In: | 2.1 |
Python’s unittest module, sometimes referred to as PyUnit, isbased on the XUnit framework design by Kent Beck and Erich Gamma. Thesame pattern is repeated in many other languages, including C, perl,Java, and Smalltalk. Beogram cd 5500 service manual. The framework implemented by unittestsupports fixtures, test suites, and a test runner to enable automatedtesting for your code.
You’ve just written one extremely clunky unit test! Introducing a Unit Test Framework. I’m sure you can pick out the flaw in this approach. While you might enjoy your newfound ability to verify that 5 + 6 does, indeed, return 11, you probably don’t want to create and run a new console project for each unit test that you. Note: If you have multiple test files with TestCase subclasses that you’d like to run, consider using python -m unittest discover to run more than one test file. Run python -m unittest discover -help for more information. Using the tearDown Method to Clean Up Resources. TestCase supports a counterpart to the setUp method named tearDown.
Basic Test Structure¶
Tests, as defined by unittest, have two parts: code to managetest “fixtures”, and the test itself. Individual tests are created bysubclassing TestCase and overriding or adding appropriatemethods. For example,
In this case, the SimplisticTest has a single test()method, which would fail if True is ever False.
Running Tests¶
The easiest way to run unittest tests is to include:
at the bottom of each test file, then simply run the script directly from thecommand line:
This abbreviated output includes the amount of time the tests took, along witha status indicator for each test (the ”.” on the first line of output meansthat a test passed). For more detailed test results, include the -v option:
Test Outcomes¶
Tests have 3 possible outcomes:
- ok
- The test passes.
- FAIL
- The test does not pass, and raises an AssertionError exception.
- ERROR
- The test raises an exception other than AssertionError.
There is no explicit way to cause a test to “pass”, so a test’s status dependson the presence (or absence) of an exception.
When a test fails or generates an error, the traceback is included in theoutput.
In the example above, testFail() fails and the traceback showsthe line with the failure code. It is up to the person reading thetest output to look at the code to figure out the semantic meaning ofthe failed test, though. To make it easier to understand the nature ofa test failure, the fail*() and assert*() methods allaccept an argument msg, which can be used to produce a more detailederror message.
Asserting Truth¶
Most tests assert the truth of some condition. There are a fewdifferent ways to write truth-checking tests, depending on theperspective of the test author and the desired outcome of the codebeing tested. If the code produces a value which can be evaluated astrue, the methods failUnless() and assertTrue() shouldbe used. If the code produces a false value, the methodsfailIf() and assertFalse() make more sense.
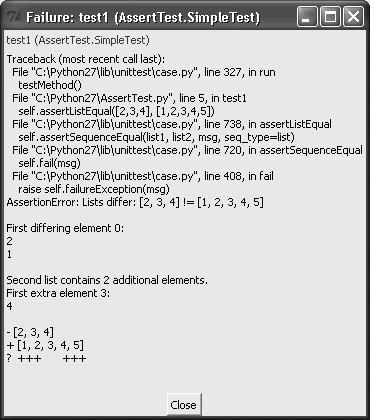
Testing Equality¶
As a special case, unittest includes methods for testing theequality of two values.
These special tests are handy, since the values being compared appearin the failure message when a test fails.
And when these tests are run:
Almost Equal?¶
In addition to strict equality, it is possible to test for nearequality of floating point numbers using failIfAlmostEqual()and failUnlessAlmostEqual().
The arguments are the values to be compared, and the number of decimalplaces to use for the test.
Testing for Exceptions¶
As previously mentioned, if a test raises an exception other thanAssertionError it is treated as anerror. This is very useful for uncovering mistakes while you aremodifying code which has existing test coverage. There arecircumstances, however, in which you want the test to verify that somecode does produce an exception. For example, if an invalid value isgiven to an attribute of an object. In such cases,failUnlessRaises() makes the code more clear than trapping theexception yourself. Compare these two tests:
The results for both are the same, but the second test usingfailUnlessRaises() is more succinct.
Test Fixtures¶

Fixtures are resources needed by a test. For example, if you arewriting several tests for the same class, those tests all need aninstance of that class to use for testing. Anu script manager 6.5. Other test fixtures includedatabase connections and temporary files (many people would argue thatusing external resources makes such tests not “unit” tests, but theyare still tests and still useful). TestCase includes aspecial hook to configure and clean up any fixtures needed by yourtests. To configure the fixtures, override setUp(). To cleanup, override tearDown().
Unit Test Runner Javascript

When this sample test is run, you can see the order of execution of thefixture and test methods:
Test Suites¶
The standard library documentation describes how to organize testsuites manually. I generally do not use test suites directly, becauseI prefer to build the suites automatically (these are automated tests,after all). Automating the construction of test suites is especiallyuseful for large code bases, in which related tests are not all in thesame place. Tools such as nose make it easier to manage tests whenthey are spread over multiple files and directories.
See also
Stackoverflow.com › Questions › 307357Best Test Runner? (Unit Testing, .NET) - Stack Overflow
- unittest
- Standard library documentation for this module.
- doctest
- An alternate means of running tests embedded in docstrings orexternal documentation files.
- nose
- A more sophisticated test manager.
- unittest2
- Ongoing improvements to unittest
.net Core - Dotnet Test Command To Display Individual Test ..
Navigation
See Full List On Github.com
- modules |
- next |
- previous |
- PyMOTW »
- Development Tools »